stepalibre
Alibre Super User
This was one of the first questions I wanted to answer when I started using Alibre Script more seriously earlier this year. Others have mentioned a need for this as well, it being an obvious feature for power users. So here I'll explain an approach to solving it. I hope it helps you. For me it saves so much time not needing to recompile the addon.

It is useful for both developing and testing addon code as well as for use as a final solution.
Reference IronPython DLLs from C:\Program Files\IronPython 2.7 or NuGet:
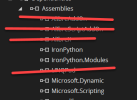
Write commands that will execute the IronPython code:
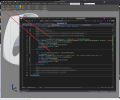
You need to load AlibreScriptAddon.dll inside of Alibre Design by launching the Alibre Script addon:
Then unload it and your Alibre Script code will run from your addon:
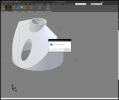
You can create any solution for loading the Alibre Script code inside your addon. Here is an example using the InputBox function to load any Alibre Script Python file:
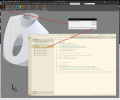
Here is an example where the code is saved to a folder inside the addon install folder. All your addon code can be Alibre Script/AlibreX Python files without any .NET code (except the command code).
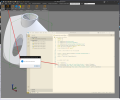
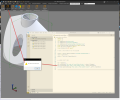
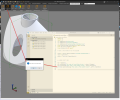
The key parts needed for the Alibre Script code to work is to reference the neccesary dlls and import modules required for Alibre Script. You can get a reference to the root and session many different ways.
Instead of enabling and disabling the Alibre Script addon you can load the required dlls in your addon.
With this approach you're using Alibre Script and AlibreX directly from Python. You don't have any of the helper functions and safety of the Alibre Script addon, much like the Alibre Script advanced API:

Existing scripts may not work the same way and need to be rewritten. My usage has mostly been simple scripts a few lines long.
This is IronPython under the hood, you could use only AlibreX, making AlibreX Python scripts.
Example Addon Command Code:
Example Alibre Script Python Script:
Example AlibreX Python Script:
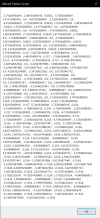
Reference IronPython DLLs from C:\Program Files\IronPython 2.7 or NuGet:
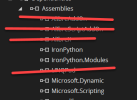
Write commands that will execute the IronPython code:
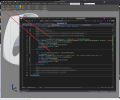
You need to load AlibreScriptAddon.dll inside of Alibre Design by launching the Alibre Script addon:
Then unload it and your Alibre Script code will run from your addon:
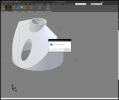
You can create any solution for loading the Alibre Script code inside your addon. Here is an example using the InputBox function to load any Alibre Script Python file:
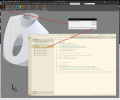
Here is an example where the code is saved to a folder inside the addon install folder. All your addon code can be Alibre Script/AlibreX Python files without any .NET code (except the command code).
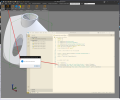
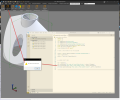
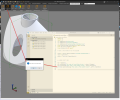
The key parts needed for the Alibre Script code to work is to reference the neccesary dlls and import modules required for Alibre Script. You can get a reference to the root and session many different ways.
Instead of enabling and disabling the Alibre Script addon you can load the required dlls in your addon.
With this approach you're using Alibre Script and AlibreX directly from Python. You don't have any of the helper functions and safety of the Alibre Script addon, much like the Alibre Script advanced API:

Existing scripts may not work the same way and need to be rewritten. My usage has mostly been simple scripts a few lines long.
This is IronPython under the hood, you could use only AlibreX, making AlibreX Python scripts.
Example Addon Command Code:
Code:
Public Function RunAddonTestFileCmd(session As IADSession) As IAlibreAddOnCommand
Dim filePath As String = Path.Combine(Path.GetDirectoryName(Assembly.GetExecutingAssembly().Location), "AlibreScripts\sample_alibre_script_000.py")
Dim eng = IronPython.Hosting.Python.CreateEngine()
Dim scope = eng.CreateScope()
Dim scriptFile = File.ReadAllText(filePath)
eng.Execute(scriptFile, scope)
Return Nothing
End Function
Public Function RunScriptFileCmd() As IAlibreAddOnCommand
Dim userInput As String
userInput = InputBox("Enter the path to a Python File:", "Run A Alibre Script Python File", "")
Dim eng = IronPython.Hosting.Python.CreateEngine()
Dim scope = eng.CreateScope()
Dim scriptFile = File.ReadAllText(userInput)
eng.Execute(scriptFile, scope)
Return Nothing
End Function
Code:
import sys
import clr
clr.AddReference("alibre-api")
clr.AddReference("AlibreScriptAddOn")
clr.AddReference('System.Runtime.InteropServices')
from System.Runtime.InteropServices import Marshal
from com.alibre.automation import *
from AlibreScript.API import *
# HERE IS ONE METHOD TO GET THE SESSION
alibre = Marshal.GetActiveObject("AlibreX.AutomationHook")
root = alibre.Root
myPart = Part(root.TopmostSession)
# ALIBRE SCRIPT CODE
Win = Windows()
Win.InfoDialog('This code is from Alibre Script.', myPart.FileName)
Win.ErrorDialog('This code is from Alibre Script!', myPart.LastAuthor)
print(Win.QuestionDialog('This code is from Alibre Script?', myPart.CreatedBy))
Code:
import sys
clr.AddReference('System.Runtime.InteropServices')
from System.Runtime.InteropServices import Marshal
alibre = Marshal.GetActiveObject("AlibreX.AutomationHook")
root = alibre.Root
session = root.TopmostSession
objADPartSession = session
b = objADPartSession.Bodies
verts = b.Item(0).Vertices
listA = []
def printpoint(x, y, z):
print("{0} , {1} , {2}".format(x, y, z))
listA.append("{0} , {1} , {2}".format(x, y, z))
for i in range(verts.Count):
vert = verts.Item(i)
point = vert.Point
printpoint(point.X, point.Y, point.Z)
from System.Windows.Forms import MessageBox, MessageBoxButtons
list_str = ', '.join(map(str, listA))
MessageBox.Show(list_str, "AlibreX Python Script", MessageBoxButtons.OK)
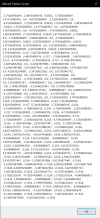
Last edited: