stepalibre
Alibre Super User
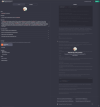
Stay tuned.

Introducing GPTs
You can now create custom versions of ChatGPT that combine instructions, extra knowledge, and any combination of skills.
openai.com
from AlibreScript import *
def create_base_plate_with_holes():
# Initialize a part session
part = CurrentPart()
# Define dimensions for the base plate and holes
plate_length = 100.0 # Length of the base plate
plate_width = 50.0 # Width of the base plate
plate_thickness = 5.0 # Thickness of the base plate
hole_diameter = 5.0 # Diameter of the holes
hole_offset = 10.0 # Offset of the holes from the edges
# Create a base plate
base_sketch = part.AddSketch('BasePlateSketch', part.XYPlane)
base_sketch.AddRectangle(-plate_width / 2, -plate_length / 2, plate_width / 2, plate_length / 2, False)
part.AddExtrudeBoss('BasePlate', base_sketch, plate_thickness, False, part.EndCondition.ToDepth, None, 0, part.DirectionType.Normal, None, 0, False)
# Create holes
for x in [-plate_width / 2 + hole_offset, plate_width / 2 - hole_offset]:
for y in [-plate_length / 2 + hole_offset, plate_length / 2 - hole_offset]:
hole_sketch = part.AddSketch('HoleSketch', part.XYPlane)
hole_sketch.AddCircle(x, y, hole_diameter / 2, False)
part.AddExtrudeCut('ThroughHole', hole_sketch, 0, False, part.EndCondition.ThroughAll, None, 0, part.DirectionType.Normal, None, 0, False)
# Save the part
#part.Save()
# Run the function to create a simple base plate with four holes
create_base_plate_with_holes()
import math
from AlibreScript import *
# Initialize the part session
part = CurrentPart()
# Assuming you have a part with an edge to chamfer.
# The edge can be obtained in various ways, depending on the context.
# Here's a generic way to get an edge, replace this with your specific method.
edges = part.GetEdges()
edge_to_chamfer = edges[0] # Assuming you want to chamfer the first edge in the list
# Define chamfer parameters
distance1 = 1 # The first chamfer distance
distance2 = 1 # The second chamfer distance (for unequal chamfer, otherwise set same as distance1)
# Add chamfer to the selected edge
part.AddChamfer("ChamferName", edge_to_chamfer, distance1, distance2, False)
# Regenerate the part to update the view
part.Regenerate()
import math
from AlibreScript import *
# Initialize the part session
part = CurrentPart()
# Define the dimensions of the bolt
hex_head_diameter = 10 # Diameter of the hex head
hex_head_height = 5 # Height of the hex head
shaft_diameter = 5 # Diameter of the shaft
shaft_length = 20 # Length of the shaft
# Create a hexagon sketch for the bolt head
hex_sketch = part.AddSketch("HexHeadSketch", part.XYPlane)
for i in range(6):
angle1 = math.radians(60 * i)
angle2 = math.radians(60 * (i + 1))
x1 = hex_head_diameter / 2 * math.cos(angle1)
y1 = hex_head_diameter / 2 * math.sin(angle1)
x2 = hex_head_diameter / 2 * math.cos(angle2)
y2 = hex_head_diameter / 2 * math.sin(angle2)
hex_sketch.AddLine([x1, y1], [x2, y2], False)
# Extrude the hex head
hex_head = part.AddExtrudeBoss("HexHead", hex_sketch, hex_head_height, False)
# Create a circle sketch for the shaft
shaft_sketch = part.AddSketch("ShaftSketch", part.XYPlane)
shaft_sketch.AddCircle(0, 0, shaft_diameter / 2, False)
# Extrude the shaft
shaft = part.AddExtrudeBoss("Shaft", shaft_sketch, shaft_length, False)
# Regenerate the part to update the view
part.Regenerate()
import math
from AlibreScript import *
# Initialize the part session
part = CurrentPart()
# Fetch the standard planes
xy_plane = part.GetPlane("XY-Plane")
zx_plane = part.GetPlane("ZX-Plane")
# Create a profile sketch on the XY plane
profile_sketch = part.AddSketch("ProfileSketch", xy_plane)
# Add a circle to the profile sketch as the sweep profile
profile_sketch.AddCircle(0, 0, 2, False) # Center at (0,0), Radius = 2
# Create a path sketch on the ZX plane
path_sketch = part.AddSketch("PathSketch", zx_plane)
# Add a line to the path sketch as the sweep path
path_sketch.AddLine([0, 0], [10, 10], False) # Line from (0,0) to (10,10)
# Define parameters for the sweep boss
is_rigid = False
end_condition = part.EndCondition.EntirePath # Using EntirePath as the end condition
end_plane = None
end_offset = 0
draft_angle = 0 # No draft angle
outward_draft = False # No outward draft
# Add the sweep boss feature
part.AddSweepBoss("SweepBossFeature", profile_sketch, path_sketch, is_rigid, end_condition, end_plane, end_offset, draft_angle, outward_draft)
# Regenerate the part to update the view
part.Regenerate()
import math
from AlibreScript import *
# Initialize the part session
part = CurrentPart()
# Flange dimensions (in mm)
flangeDiameter = 565 # Flange outer diameter
flangeThickness = 30 # Flange thickness
pipeDiameter = 254 # 10 inches converted to mm for pipe
boltHoleDiameter = 40 # Bolt hole diameter
boltCircleDiameter = 175 # Bolt circle diameter
numberOfBoltHoles = 12
# Create main flange disc
flangeSketch = part.AddSketch('FlangeBase', part.XYPlane)
flangeSketch.StartEditing()
flangeSketch.AddCircle(0, 0, flangeDiameter / 2, False) # Center at (0,0)
flangeSketch.StopEditing()
flange = part.AddExtrudeBoss('FlangeDisc', flangeSketch, flangeThickness, False)
# Create bolt holes
for i in range(numberOfBoltHoles):
angle = 2 * math.pi * i / numberOfBoltHoles
x = boltCircleDiameter / 2 * math.cos(angle)
y = boltCircleDiameter / 2 * math.sin(angle)
boltHoleSketch = part.AddSketch('BoltHole', part.XYPlane)
boltHoleSketch.StartEditing()
boltHoleSketch.AddCircle(x, y, boltHoleDiameter / 2, False)
boltHoleSketch.StopEditing()
part.AddExtrudeCut('BoltHoleCut', boltHoleSketch, flangeThickness, False)
# Create center pipe hole
centerHoleSketch = part.AddSketch('CenterPipeHole', part.XYPlane)
centerHoleSketch.StartEditing()
centerHoleSketch.AddCircle(0, 0, pipeDiameter / 2, False)
centerHoleSketch.StopEditing()
part.AddExtrudeCut('CenterHoleCut', centerHoleSketch, flangeThickness, False)
print("10-inch pipe flange model created.")
import math
from AlibreScript import *
# Initialize the part session
part = CurrentPart()
# Nut dimensions (in mm)
threadDiameter = 10 # M10 thread
pitch = 1.5 # Typical pitch for M10
acrossFlats = 17 # Width across flats for M10
thickness = 8 # Nut thickness for M10
cornerRadius = 1 # Corner radius
# Number of sides for the hexagon
numSides = 6
# Create the base hexagonal profile
hexSketch = part.AddSketch('HexBase', part.XYPlane)
hexSketch.StartEditing()
# Calculate vertices for the hexagon
vertices = []
for i in range(numSides + 1): # +1 to close the hexagon
angle = 2 * math.pi * i / numSides
x = acrossFlats / (2 * math.cos(math.pi / numSides)) * math.cos(angle)
y = acrossFlats / (2 * math.cos(math.pi / numSides)) * math.sin(angle)
vertices.append([x, y])
# Add lines to create the hexagon
for i in range(numSides):
startX, startY = vertices[i]
endX, endY = vertices[i + 1]
hexSketch.AddLine(startX, startY, endX, endY, False) # Adding line using coordinates
hexSketch.StopEditing()
# Extrude the hexagon to form the nut body
nutBody = part.AddExtrudeBoss('NutBody', hexSketch, thickness, False)
# Create internal thread hole
threadHoleSketch = part.AddSketch('ThreadHole', part.XYPlane)
threadHoleSketch.StartEditing()
threadHoleSketch.AddCircle(0, 0, threadDiameter / 2, False)
threadHoleSketch.StopEditing()
part.AddExtrudeCut('ThreadHoleCut', threadHoleSketch, thickness, False)
print("M10 hex nut model created.")
from __future__ import division
to fix integer division in ironpython 2.7 or for example 5/2 will equal 2 instead of 2.5hole_sketch.AddCircle(x, y, hole_diameter / 2, False)
should be hole_sketch.AddCircle(x, y, hole_diameter, False)
as the AddCircle expects a diameter not a radius.Units.Current = UnitTypes.Millimeters
Units.Current = UnitTypes.Centimeters
Units.Current = UnitTypes.Inches
I will add this to my updates when I revisit it next year. Any more comments and thoughts would be appreciated.Might want to check those again. The results are not all matching the input numbers.
Most importantly the top of each script with any math it should includefrom __future__ import division
to fix integer division in ironpython 2.7 or for example 5/2 will equal 2 instead of 2.5
In the first onehole_sketch.AddCircle(x, y, hole_diameter / 2, False)
should behole_sketch.AddCircle(x, y, hole_diameter, False)
as the AddCircle expects a diameter not a radius.
Basic hex head bolt code has the same AddCircle and division problem and also since both extrusions are going the same direction from the same face the bolt shaft ends up being too short.
AddSweepBoss has same AddCircle issues. I think I found a bug in AlibreScript when testing a draft angle - it converts it to radians when it should not (or maybe twice?).
10 pipe flange model has the same AddCircle and division problems and bogus flange data - boltCircleDiameter can not be smaller than pipeDiameter or the bolt holes all get cut off.
I'd suggest adding all holes to one sketch and doing a single extrude cut to keep the feature history shorter. Hopefully Alibre will add the ability to add patterns using API / AlibreScript soon.
The M10 hex nut has the same AddCircle and division problems. The pitch and cornerRadius variables are not used.
I'd also suggest also includingUnits.Current = UnitTypes.Millimeters
orUnits.Current = UnitTypes.Centimeters
orUnits.Current = UnitTypes.Inches
so users can easily see a default and where to change it.
#https://help.alibre.com/articles/#!alibre-help-v23/triangle
# Create triangle with angles 90, 15, 75
import math
# set up parameters
Theta = 15.0
Adjacent = 100.0
# calculate side
Opposite = Adjacent * math.tan(math.radians(Theta))
# generate three vertices of triangle
P1_X = 0
P1_Y = 0
P2_X = Adjacent
P2_Y = 0
P3_X = Adjacent
P3_Y = Opposite
# create part and sketch
P = Part('Foo')
S = P.AddSketch('Shape', P.GetPlane('XY-Plane'))
# draw it
S.AddLine(P1_X, P1_Y, P2_X, P2_Y, False)
S.AddLine(P2_X, P2_Y, P3_X, P3_Y, False)
S.AddLine(P3_X, P3_Y, P1_X, P1_Y, False)
import math
def create_right_triangle(theta, adjacent_length, part_name, sketch_name, plane_name):
# Calculate the length of the opposite side using trigonometry
opposite_length = adjacent_length * math.tan(math.radians(theta))
# Define the vertices of the triangle
p1 = (0, 0)
p2 = (adjacent_length, 0)
p3 = (adjacent_length, opposite_length)
# Create a new part and a sketch on the specified plane
part = Part(part_name)
sketch = part.AddSketch(sketch_name, part.GetPlane(plane_name))
# Draw the triangle using lines between the vertices
sketch.AddLine(p1[0], p1[1], p2[0], p2[1], False)
sketch.AddLine(p2[0], p2[1], p3[0], p3[1], False)
sketch.AddLine(p3[0], p3[1], p1[0], p1[1], False)
# Set up parameters for the triangle
theta = 15.0 # Angle in degrees
adjacent_length = 100.0 # Length of the adjacent side
# Create the triangle
create_right_triangle(theta, adjacent_length, 'TrianglePart', 'TriangleSketch', 'XY-Plane')
import sys
import math
Win = Windows()
Options = []
Options.append(['Radius', WindowsInputTypes.Real, 10.0])
Options.append(['Pitch', WindowsInputTypes.Real, 5.0])
Options.append(['Turns', WindowsInputTypes.Integer, 10])
Options.append(['Angle Increment', WindowsInputTypes.Real, 0.1])
Values = Win.OptionsDialog('Helix Parameters', Options)
if Values == None:
sys.exit('User cancelled')
Radius = Values[0]
Pitch = Values[1]
Turns = Values[2]
AngleIncrement = Values[3]
print('Radius = %f' % Radius)
print('Pitch = %f' % Pitch)
print('Turns = %d' % Turns)
print('Angle Increment = %f' % AngleIncrement)
Points = []
Angle = 0.0
HeightIncrement = Pitch / (2 * math.pi / AngleIncrement)
for Pass in range(int(Turns * (2 * math.pi / AngleIncrement))):
X = Radius * math.cos(Angle)
Y = Radius * math.sin(Angle)
Z = Pass * HeightIncrement
Points.extend([X, Y, Z])
Angle += AngleIncrement
Helix = CurrentPart()
Path = Helix.Add3DSketch('Path')
Path.AddBspline(Points)
import sys
import math
Win = Windows()
Options = []
Options.append(['Angle Increment', WindowsInputTypes.Real, 0.05])
Options.append(['Radius', WindowsInputTypes.Real, 1.0])
Options.append(['Height Increment', WindowsInputTypes.Real, 0.1])
Options.append(['Turns', WindowsInputTypes.Integer, 20])
Values = Win.OptionsDialog('Helix Parameters', Options)
if Values is None:
sys.exit('User cancelled')
AngleIncrement = Values[0]
Radius = Values[1]
HeightIncrement = Values[2]
Turns = Values[3]
print('Angle Increment = %f' % AngleIncrement)
print('Radius = %f' % Radius)
print('Height Increment = %f' % HeightIncrement)
print('Turns = %d' % Turns)
Points = []
Angle = 0.0
Height = 0.0
TotalAngle = 2 * math.pi * Turns
while Angle <= TotalAngle:
X = Radius * math.cos(Angle)
Y = Radius * math.sin(Angle)
Z = Height
Points.extend([X, Y, Z])
Angle += AngleIncrement
Height += HeightIncrement
HelixPart = Part('Helix')
Path = HelixPart.Add3DSketch('Path')
Path.AddBspline(Points)
import sys
import math
Win = Windows()
Options = []
Options.append(['Angle Increment', WindowsInputTypes.Real, 0.05])
Options.append(['Radius', WindowsInputTypes.Real, 1.0])
Options.append(['Height Increment', WindowsInputTypes.Real, 0.1])
Options.append(['Turns', WindowsInputTypes.Integer, 20])
Values = Win.OptionsDialog('Helix Parameters', Options)
if Values is None:
sys.exit('User cancelled')
AngleIncrement = Values[0]
Radius = Values[1]
HeightIncrement = Values[2]
Turns = Values[3]
Points = []
Angle = 0.0
Height = 0.0
TotalAngle = 2 * math.pi * Turns
while Angle <= TotalAngle:
X = Radius * math.cos(Angle)
Y = Radius * math.sin(Angle)
Z = Height
Points.extend([X, Y, Z])
Angle += AngleIncrement
Height += HeightIncrement * AngleIncrement / (2 * math.pi)
HelixPart = Part('Helix')
Path = HelixPart.Add3DSketch('Path')
Path.AddBspline(Points)
import sys
import math
Win = Windows()
Options = []
Options.append(['Radius', WindowsInputTypes.Real, 10.0])
Options.append(['Pitch', WindowsInputTypes.Real, 5.0])
Options.append(['Total Turns', WindowsInputTypes.Integer, 10])
Options.append(['Angle Increment', WindowsInputTypes.Real, 0.1])
Options.append(['Segment Angle', WindowsInputTypes.Integer, 30]) # Option for segment angle
Values = Win.OptionsDialog('Helix Parameters', Options)
if Values is None:
sys.exit('User cancelled')
Radius = Values[0]
Pitch = Values[1]
TotalTurns = Values[2]
AngleIncrement = Values[3]
SegmentAngle = Values[4] # Angle for each segment
print('Radius = %f' % Radius)
print('Pitch = %f' % Pitch)
print('Total Turns = %d' % TotalTurns)
print('Angle Increment = %f' % AngleIncrement)
print('Segment Angle = %d' % SegmentAngle)
# Calculate the total number of segments based on total turns and segment angle
Segments = int((TotalTurns * 360) / SegmentAngle)
for Segment in range(Segments):
Points = []
# Calculate the start and end angles for the current segment in radians
StartAngle = Segment * SegmentAngle * (math.pi / 180.0)
EndAngle = StartAngle + (SegmentAngle * (math.pi / 180.0))
# Adjust the starting Z coordinate based on the segment's position in the overall helix
StartZ = Pitch * (StartAngle / (2 * math.pi))
Angle = StartAngle
while Angle < EndAngle:
X = Radius * math.cos(Angle)
Y = Radius * math.sin(Angle)
# Calculate Z using the adjusted start Z for the segment and the angle's progression
Z = StartZ + Pitch * ((Angle - StartAngle) / (2 * math.pi))
Points.extend([X, Y, Z])
Angle += AngleIncrement * (math.pi / 180.0) # Convert angle increment to radians for consistent progression
# Assuming CurrentPart() and Add3DSketch are part of your CAD API
Helix = CurrentPart()
PathName = 'Path_Segment_%d' % (Segment + 1)
Path = Helix.Add3DSketch(PathName)
Path.AddBspline(Points)