LuminescentLoom
Member
I can't make sense of what's happening here. When an assembly has a 1st-level subassembly that's suppressed, then I can find it in the collection returned by:
and it has
But if there's a 2nd-level subassembly that's suppressed, it doesn't appear in .Occurrences() at all. However, it does appear in IADDesignSession.ConstituentPaths().
The problem this causes is I'm using
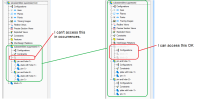
IADAssemblySession.RootOccurrence.Occurrences()
and it has
.IsSuppressed = True
as expected.But if there's a 2nd-level subassembly that's suppressed, it doesn't appear in .Occurrences() at all. However, it does appear in IADDesignSession.ConstituentPaths().
The problem this causes is I'm using
IADDesignSession.ConstituentPaths()
to look for missing files, since they don't appear in Occurrences()
, but when there's a suppressed sub-sub-assembly, I can't distinguish it from a missing file.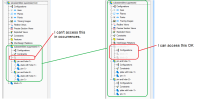