ajayre
Alibre Super User
This tutorial demonstrates the new Alibre Script sketch mapping feature in Alibre Design 2019. The primary use is when writing scripts that modify existing parts making it easier to create 2D sketches without having to get into complex calculations. A description of the functionality can also be found in the Alibre Script manual.
We will start off with a part that was created elsewhere, e.g. manually, provided to us, etc. For this example we use a hexagonal extrusion that gets larger towards the top. Diameter of bottom hexagon is 30mm, diameter of top hexagon is 45mm and the height is 70mm.
Our script will create a notch in the top. The script lets the user choose the face to notch and which edge is the top. If we manually create a 2D sketch on the face we can see the problem.
I have drawn the X and Y axes on top of the face using reference lines. We don't know in advance exactly how the face will be positioned in the 2D space. As it happens one edge is parallel to the X axis which means that the top edge is 86.48 degrees from vertical.
In order to create a rectangle in the sketch for notch we now have to use trigonometry to calculate the four corners. Here is what the final sketch should look like (drawn manually)
Namely a rectangle 10mm x 2mm centered on the top edge. Who wants to do a load of calculations just to draw a rectangle? Nobody. This is where sketch mapping comes in.
We will start off with a part that was created elsewhere, e.g. manually, provided to us, etc. For this example we use a hexagonal extrusion that gets larger towards the top. Diameter of bottom hexagon is 30mm, diameter of top hexagon is 45mm and the height is 70mm.
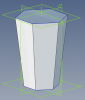
Our script will create a notch in the top. The script lets the user choose the face to notch and which edge is the top. If we manually create a 2D sketch on the face we can see the problem.
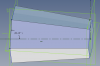
I have drawn the X and Y axes on top of the face using reference lines. We don't know in advance exactly how the face will be positioned in the 2D space. As it happens one edge is parallel to the X axis which means that the top edge is 86.48 degrees from vertical.
In order to create a rectangle in the sketch for notch we now have to use trigonometry to calculate the four corners. Here is what the final sketch should look like (drawn manually)
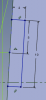
Namely a rectangle 10mm x 2mm centered on the top edge. Who wants to do a load of calculations just to draw a rectangle? Nobody. This is where sketch mapping comes in.